출처 : https://javascript.info/basic-dom-node-properties
DOM node classes
다른 DOM nodes는 다른 properties를 갖고 있다. 예를들어 <a> tag에 해당하는 element node는 link와 연관된 properties를 갖는다. 하지만, 공통 properties와 함수도 있다. DOM nodes의 모든 class는 하나의 hierarchy에서 나왔기 때문이다.
hierarchy의 근본은 EventTarget이며 Node에 의해 상속된다.
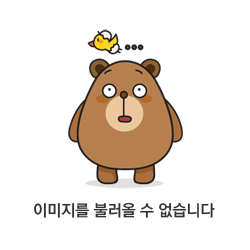
- EventTarget - root 'abstract' class, 근본 추상 클래스, 따라서 모든 DOM nodes는 'events'를 지원한다.
- Node - 또한 추상 클래스, core tree 기능(parentNode, nextSibling, childNodes...)을 제공한다.
- Document
- CharacterData - 추상 클래스
- Text
- Comment
- Element - element-level navigation(nextElementSibling, children), searching 함수(getElementsByTagName, querySelector)를 제공한다.
- HTMLElement - 모든 HTML elements의 basic class이다.
예를들어 <input> element의 DOM object를 살펴보자.
상속 순서에 따라 properties를 확인할 수 있다.
- HTMLInputElement
- HTMLElement
- Element
- Node
- EventTarget
- Object
DOM node class name을 확인하기 위해 Object의 constructor property를 호출한다.
alert( document.body.constructor.name ); // HTMLBodyElement
또는 단지 toString할 수 있다.
alert( document.body ); // [object HTMLBodyElement]
상속여부를 확인하기 위해 instanceof를 사용할 수도 있다.
alert( document.body instanceof HTMLBodyElement ); // true
alert( document.body instanceof HTMLElement ); // true
alert( document.body instanceof Element ); // true
alert( document.body instanceof Node ); // true
alert( document.body instanceof EventTarget ); // true
DOM nodes는 일반적인 JavaScript objects이며, 상속을 위해 prototype기반 class를 사용한다.
console.dir(elem)을 실행하면, console에서 HTMLElement.prototype, Element.prototype등을 볼 수 있다.
console.dir(elem) vs console.log(elem)
대부분의 browsers에서 console.log, console.dir을 지원한다.
- console.log(elem) : element DOM tree를 보여준다.
- console.dir(elem) : DOM object로 element를 보여준다. properties를 확인하기 좋다.
<!DOCTYPE html>
<html lang="en">
<head>
</head>
<body>
<script>
console.log(document.body);
console.dir(document.body);
</script>
</body>
</html>
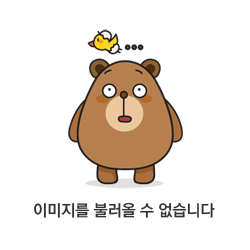
The “nodeType” property
DOM node type을 확인할 수 있지만, 요즘엔 instanceof를 사용한다.
Tag: nodeName and tagName
alert( document.body.nodeName ); // BODY
alert( document.body.tagName ); // BODY
tagName과 nodeName의 차이점은?
tagName은 Element nodes에만 존재하고, nodeName은 어느 Node에나 정의되어 있다.
<body><!-- comment -->
<script>
// for comment
alert( document.body.firstChild.tagName ); // undefined (not an element)
alert( document.body.firstChild.nodeName ); // #comment
// for document
alert( document.tagName ); // undefined (not an element)
alert( document.nodeName ); // #document
</script>
</body>
element에 대해서는 tagName과 nodeName의 차이점은 없다.
innerHTML: the contents
innerHTML property는 element 내부의 HTML을 string으로 반환하며, 수정도 가능하다.
innerHTML이 document내부로 <script> tag를 추가하는 경우, HTML의 일부분이 되지만, script가 실행되지는 않는다.
Beware: “innerHTML+=” does a full overwrite
elem.innerHTML+="more html"을 사용하여 element를 HTML에 append할 수 있다.
하지만, 해당 코드는 조심해야 사용해야 한다. 왜냐하면 실행되는 방법이 addition이 아닌 full overwrite이기 때문이다.
따라서, 기술적으로 아래 두 라인은 동일하다.
elem.innerHTML += "...";
// is a shorter way to write:
elem.innerHTML = elem.innerHTML + "..."
chatDiv.innerHTML += "<div>Hello<img src='smile.gif'/> !</div>";
chatDiv.innerHTML += "How goes?"; //HTML content를 재생성하고, smile.gif를 reload한다.
//만약에 chatDiv가 많은 양의 text와 images를 갖고 있다면 reload는 시각적으로 보여질 수 있다.
outerHTML: full HTML of the element
outerHTML은 element의 full HTML이다. innerHTML에 element자신을 더한 것이다.
innerHTML과 다르게, outerHTML은 DOM element를 수정하지 않는다. 대신에 DOM에서 교체될 수 있다.
<div>Hello, world!</div>
<script>
let div = document.querySelector('div');
// div가 document에서 제거된다.
//<p>...</p>가 그 자리에 insert된다.
div.outerHTML = '<p>A new element</p>'; // (*)
// div는 예전값을 그대로 가지고 있다. new HTML은 어느 변수에도 저장되지 않았다.
alert(div.outerHTML); // <div>Hello, world!</div> (**)
</script>
nodeValue/data: text node content
innerHTML property는 element node에서만 유효하며, text nodes와 같은 다른 node types에서는 nodeValue, data properties를 사용할 수 있다.
<body>
Hello
<!-- Comment -->
<script>
let text = document.body.firstChild;
alert(text.data); // Hello
let comment = text.nextSibling;
alert(comment.data); // Comment
</script>
</body>
textContent: pure text
<div id="news">
<h1>Headline!</h1>
<p>Martians attack people!</p>
</div>
<script>
// Headline! Martians attack people!
alert(news.textContent);
</script>
text만 return되며, 모든 tag는 제외된다.
안전한 방법으로 text를 쓰기 위해서는 textContent가 유용하다.
- innerHTML : HTML으로 추가된다.
- textContent : text로 추가된다.
<div id="elem1"></div>
<div id="elem2"></div>
<script>
let name = prompt("What's your name?", "<b>Winnie-the-Pooh!</b>");
document.getElementById('elem1').innerHTML = name; //tags가 되므로 bold name을 볼 수 있다.
document.getElementById('elem2').textContent = name; //<b>Winnie-the-Pooh!</b> 텍스트를 볼 수 있다.
</script>
The “hidden” property
<div>Both divs below are hidden</div>
<div hidden>With the attribute "hidden"</div>
<div id="elem">JavaScript assigned the property "hidden"</div>
<script>
document.getElementById('elem').hidden = true;
</script>
hidden은 style="display:none"과 동일하게 동작한다.
More properties
DOM elements는 class에 따라 추가적인 properties를 갖고 있다.
- value - HTMLInputElement
- href - HTMLAnchorElement
- id - HTMLElement
<input type="text" id="elem" value="value">
<script>
alert(document.getElementById('elem').type); // "text"
alert(document.getElementById('elem').id); // "elem"
alert(document.getElementById('elem').value); // value
</script>
브라우저 개발도구에서 Elements tab에서 DOM Properties전체 확인이 가능하다.
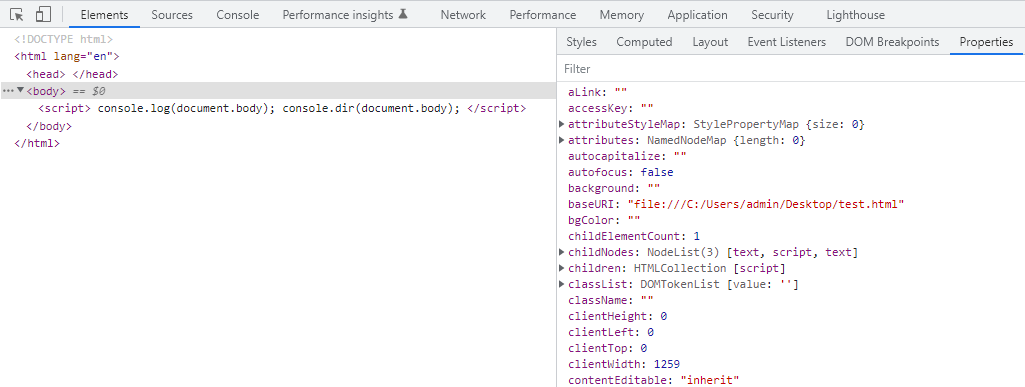
Summary
각 DOM node는 특정 class에 소속되어 있다. DOM node의 주요 properties는 아래와 같다.
- nodeType : text 또는 element node인지 볼 수 있다. numeric value를 가지고 있다. 1은 element, 3은 text이며 Read-only이다.
- nodeName/tagName : elements에는 tagName을 사용하여 non-elements에는 nodeName을 사용한다.
- innerHTML
- outerHTM;
- nodeValue/data
- textContent
- hidden
대부분의 HTML attributes는 해당하는 DOM property가 있다. 하지만 항상 같은 것은 아니다.
'JavaScript' 카테고리의 다른 글
JavaScript] document 수정 (0) | 2022.11.11 |
---|---|
JavaScript] HTML attributes and DOM properties (0) | 2022.11.08 |
JavaScript] Searching: getElement*, querySelector* (0) | 2022.11.04 |
JavaScript] DOM Navigation (0) | 2022.11.03 |
JavaScript] DOM tree (0) | 2022.11.03 |
댓글