출처 : https://javascript.info/dom-navigation
원하는 element를 수정하기 위해서는 해당하는 DOM object가 필요하다. DOM의 모든 동작은 document object와 시작한다. 이로부터 어떠한 node에도 접근할 수 있다.
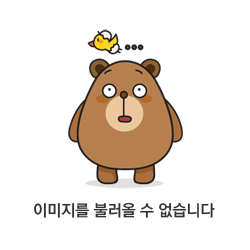
On top: documentElement and body
최상단 3개의 document properties가 있다.
<html> = document.documentElement
<body> = document.body
<head> = docuement.head
document.body는 null일 수 있다.
<html>
<head>
<script>
alert( "From HEAD: " + document.body ); // null, there's no <body> yet
</script>
</head>
<body>
<script>
alert( "From BODY: " + document.body ); // HTMLBodyElement, now it exists
</script>
</body>
</html>
Children: childNodes[nodenumber], firstChild, lastChild
- Child nodes (or children) - direct children인 elements. 예를들어 <head>, <body>는 <html> element의 children이다.
- Descendants - tag안에 포함된 모든 elements이다. children의 children까지 모두 포함.
childNodes collection은 text nodes를 포함한 모든 child nodes이다.
<html>
<body>
<div>Begin</div>
<ul>
<li>Information</li>
</ul>
<div>End</div>
<script>
for (let i = 0; i < document.body.childNodes.length; i++) {
alert( document.body.childNodes[i] ); // Text, DIV, Text, UL, ..., SCRIPT
}
</script>
...more stuff...
</body>
</html>
위의 소스에서 script가 실행되는 시점에 more stuff를 browser가 읽을 수 없기 때문에 last element가 SCRIPT가 된다.
firstChild, lastChild는 빠른 접근을 제공한다.
child nodes가 존재하면 아래 소스는 항상 true이다. child nodes의 존재여부를 체크하는 elem.hasChildNodes() 함수도 있다.
elem.childNodes[0] === elem.firstChild
elem.childNodes[elem.childNodes.length - 1] === elem.lastChild
DOM collections
childNodes는 iterable한 collection이다. DOM collections는 read-only이다.
for (let node of document.body.childNodes) {
alert(node); // shows all nodes from the collection
}
Siblings and the parent
Siblings : 부모가 같은 children
예를들어 <head>와 <body>는 siblings이다.
- <body>는 <head>의 next 또는 right sibling이다.
- <head>는 <body>의 previous 또는 left sibling이다.
// parent of <body> is <html>
alert( document.body.parentNode === document.documentElement ); // true
// after <head> goes <body>
alert( document.head.nextSibling ); // HTMLBodyElement
// before <body> goes <head>
alert( document.body.previousSibling ); // HTMLHeadElement
Element-only navigation
Navigation properties는 모든 nodes를 나타낸다. 예를들어 childNodes안에는 text nodes, elements nodes, comment nodes도 볼 수 있다.
많은 업무에서 우리는 text 또는 comment nodes는 필요하지 않다.
elements node만 계산하는 navigation link를 살펴보자.
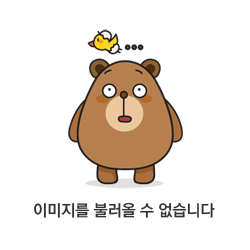
- children – only those children that are element nodes.
- firstElementChild, lastElementChild – first and last element children.
- previousElementSibling, nextElementSibling – neighbor elements.
- parentElement – parent element.
※ 왜 parentElement인가? parent가 element가 아닐 수도 있는가?
parentNodes는 'any node'도 반환할 수 있는 반면, parentElement는 'element'를 반환한다.
alert( document.documentElement.parentNode ); // document
alert( document.documentElement.parentElement ); // null
root node인 document.documentElement (<html>)은 부모로 document를 갖는다. 하지만 document는 element node가 아니다.
위의 예 중에서 childNodes를 children으로 대체한다. 이제 elements만 보여준다.
<html>
<body>
<div>Begin</div>
<ul>
<li>Information</li>
</ul>
<div>End</div>
<script>
for (let elem of document.body.children) {
alert(elem); // DIV, UL, DIV, SCRIPT
}
</script>
...
</body>
</html>
More links: tables
DOM elements의 특정 types은 편의상 추가적인 properties를 제공한다.
Tables이 그 예이다.
<table> element는 다음의 properties를 제공한다.
- table.rows – the collection of <tr> elements of the table.
- table.caption/tHead/tFoot – references to elements <caption>, <thead>, <tfoot>.
- table.tBodies – the collection of <tbody> elements (can be many according to the standard, but there will always be at least one – even if it is not in the source HTML, the browser will put it in the DOM).
<thead>, <tfoot>, <tbody> element는 다음의 row property를 제공한다.
- tbody.rows – the collection of <tr> inside.
<tr>:
- tr.cells – the collection of <td> and <th> cells inside the given <tr>.
- tr.sectionRowIndex – the position (index) of the given <tr> inside the enclosing <thead>/<tbody>/<tfoot>.
- tr.rowIndex – the number of the <tr> in the table as a whole (including all table rows).
<td> and <th>:
- td.cellIndex – the number of the cell inside the enclosing <tr>.
<table id="table">
<tr>
<td>one</td><td>two</td>
</tr>
<tr>
<td>three</td><td>four</td>
</tr>
</table>
<script>
// get td with "two" (first row, second column)
let td = table.rows[0].cells[1];
td.style.backgroundColor = "red"; // highlight it
</script>
Summary
주어진 DOM node에서 navigation properties를 이용해 이웃 node로 접근할 수 있다.
- For all nodes: parentNode, childNodes, firstChild, lastChild, previousSibling, nextSibling.
- For element nodes only: parentElement, children, firstElementChild, lastElementChild, previousElementSibling, nextElementSibling.
'JavaScript' 카테고리의 다른 글
JavaScript] DOM Node properties (0) | 2022.11.04 |
---|---|
JavaScript] Searching: getElement*, querySelector* (0) | 2022.11.04 |
JavaScript] DOM tree (0) | 2022.11.03 |
JavaScript] Browser environment, DOM, BOM (0) | 2022.11.03 |
JavaScript] 웹에서 Apple Login 구성하기 (2) | 2021.10.13 |
댓글